Time Loops
Understanding Time Complexities and Time Loops
Time Complexity in Algorithm Analysis
Time complexity is a crucial concept in algorithm analysis that helps us understand how the running time of an algorithm grows as the input size increases. It provides a theoretical estimate of the number of operations an algorithm will perform based on the input size.
Common Time Complexities
Here are some common time complexities you may encounter:
- O(1) - Constant Time: Operations take a constant time regardless of the input size.
- O(log n) - Logarithmic Time: Example is binary search where the input size decreases by a constant factor in each step.
- O(n) - Linear Time: The running time increases linearly with the input size.
- O(n^2) - Quadratic Time: Common in nested loops where the running time grows quadratically.
- O(2^n) - Exponential Time: Running time doubles with each addition to the input.
Optimizing Time Complexity
To improve the efficiency of algorithms, it's essential to optimize their time complexity. This can involve algorithm redesign, data structure selection, or applying more efficient techniques.
Understanding Time Loops
In programming, time loops refer to situations where the time complexity of an algorithm is directly related to the number of iterations or repetitions in a loop structure. Nested loops are common culprits for increasing time complexity exponentially.
Example of Time Loops
Consider the following example of a nested loop:
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
// Some operation here
}
}
In this case, the time complexity is O(n^2) as the operations will run n * n times.
Conclusion
Understanding time complexities and being mindful of time loops is crucial for writing efficient algorithms and improving the performance of your code. By analyzing and optimizing time complexities, you can make your algorithms more scalable and effective.
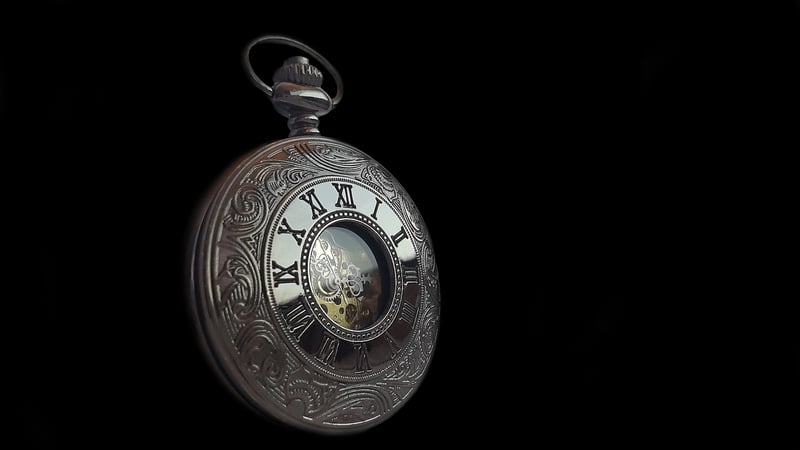
For further reading on time complexities and algorithm analysis, you can refer to resources like Wikipedia - Time Complexity and online courses on algorithms and data structures.